Yosmany Garcia
yosmanyga@gmail.com
Las Vegas, NV
Software craftsman with experience in web and mobile development using leading edge technologies.
Education
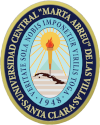
Central University of Las Villas, Cuba
Bachelor of Computer Science.
Skills
Present:
- Typescript, Reactjs, Styled-components, MobX, Inversify
- React Native, Expo
- PHP, Symfony
- Nx, Ubuntu, Docker, WebStorm, PHPStorm
Past:
- Node.js, Angular, Backbone.js, Ember.js
- Bootstrap, MUI, Sencha Ext JS, Yahoo YUI, CSS YAML
- Laravel, CakePHP, CodeIgniter, WACT
- MySQL, PostgreSQL, SQLite
- WordPress, Drupal, Joomla, eZ Publish, Expression Engine
- Notepad++, Macromedia Dreamweaver, Macromedia Fireworks, WAMP Server, Vagrant, Windows, Debian
- Turbo Pascal, Quick BASIC, Microsoft BASIC
Portfolio

The Fortress: Inside The Beast's guts
A Role Played Game (RPG) as a text adventure.
"You are common mortal who has received a letter which states you've been chosen to infiltrate The Fortress and kill the Beast, a mysterious and fearsome creature."
It's based on the original MS-DOS version, by Miguel Cepero from Merchise Group, Central University of Las Villas, in the nineties. It was a very popular game in Cuba.
Web development stack:
- The API was built with PHP.
- The database used was MongoDB.
- The development followed Behavior-driven approach using Behat.
- The security was implemented using JSON Web Token (RFC 7519).
- Received help for bored stuff from GitHub Copilot. and Chat GPT.
- The frontend was implemented using Typescript.
- The framework used was React.
- The state management was implemented using Mobx.
- The architecture design was done object-oriented, with a service container, using Inversify.
- The navigation was implemented using React Router.
- The styling was implemented using Styled-components as CSS-in-JS solution.
- The unit testing was implemented using Jest.
- The playground used for UI components was Storybook.
Mobile development stack:
- All the business logic from the web version, was reused for the mobile, such as state classes, API, and UI components as well.
- The framework used was React Native
- Expo was used as the toolkit around React Native
- The styling was implemented using Styled-components Native
- The navigation was implemented using React Navigation.
Web Trailer:
Mobile Trailer:
Validation
A library for validating simple values, arrays and objects.
// Validates that the value is an integer and is greater than 10
$validator = new ScalarValidator(array(
'type' => 'integer',
'gt' => 10
));
$errors = $validator->validate(9);
foreach ($errors as $error) {
echo $error;
echo "\r\n";
}
// Validates that the value is greater than 1 and lower than 10
// "value" will be replaced by given value
$validator = new ExpressionScalarValidator(array(
'expression' => 'value > 1 and value < 10'
));
$errors = $validator->validate(11);
// Validates that a value is an array and keys must fit these requirements:
// key1 and key2 are required
// key3 is optional
// key4 is denied
$validator = new ArrayValidator(array(
'requiredKeys' => array('key1', 'key2'),
'allowedKeys' => array('key3'),
'deniedKeys' => array('key4')
));
$errors = $validator->validate(array('key1' => 'foo1'));
$user = new User();
$user->name = 'John';
$user->age = 30;
// Validates that the property 'name' is a string
// and the property 'age' is an integer and is greater than 0
$validator = new ObjectValidator(array(
'name' => new ScalarValidator(array(
'type' => 'string'
)),
'age' => new ScalarValidator(array(
'type' => 'integer'
'gt' => 0
))
));
$errors = $validator->validate($user);
For more details see full documentation.
Resource
A library for defining and loading resources.
// A Resource is an object containing metadata that refers to something like a file,
// a directory, a db table or anything else with data:
$resource = new Resource(array(
'file' => '/path/to/a/file'
));
// You can read a resource by using a Reader. The read is done usually iterating over the data at first level:
/* resource.yml:
item1:
item11: value11
item12: value12
item2:
item22: value22
*/
$reader = new YamlFileReader();
$reader->open($resource);
while ($item = $reader->current()) {
print_r($item);
$reader->next();
}
$reader->close();
/* Output
Array
(
[key] => item1
[value] => Array
(
[item11] => value11
[item12] => value12
)
)
Array
(
[key] => item2
[value] => Array
(
[item22] => value22
)
)
*/
// Each Reader returns the data as an array but with it respective format.
// That's why you should use a Normalizer to normalize the data after read it:
$reader = new YamlFileReader();
$normalizer = new MyDataYamlFileNormalizer();
$reader->open($resource);
while ($item = $reader->current()) {
$item = $normalizer->normalize($item, $resource);
print_r($item);
$reader->next();
}
$reader->close();
// In the same way you use a DelegatorReader you can use a DelegatorNormalizer:
$normalizer = new DelegatorNormalizer(array(
new MyDataYamlFileNormalizer(),
new MyDataXmlFileNormalizer(),
new MyDataIniFileNormalizer(),
new MyDataAnnotationFileNormalizer()
));
// Keep in mind that you can use the same readers in different places,
// but you should implement custom normalizers for your data.
// Your normalizers should return data in the same format, maybe an array, or an object, that's your choice.
// You can implement all the workflow inside a Loader:
class Loader implements LoaderInterface
{
/**
* @var ReaderInterface
*/
private $reader;
/**
* @var NormalizerInterface
*/
private $normalizer;
/**
* @var CacherInterface
*/
private $cacher;
public function __construct(
ReaderInterface $reader,
NormalizerInterface $normalizer,
CacherInterface $cacher)
{
$this->reader = $reader;
$this->normalizer = $normalizer;
$this->cacher = $cacher;
}
/**
* @param $resource Resource
* @return array
*/
public function load($resource)
{
if ($this->cacher->check($resource)) {
return $this->cacher->retrieve($resource);
}
$this->reader->open($resource);
$items = array();
while ($item = $this->reader->current()) {
$items[] = $this->normalizer->normalize($item, $resource);
$this->reader->next();
}
$reader->close();
$this->cacher->store($items, $resource);
return $items;
}
}
For more details see full documentation.